Structured Query Language 1
Since 334 has to and likes to play with DATA, learning DB is essential.

Relational database management system
Intro
Database: A collection of associated tables.
Table: A matrix of data.
Redundancy: Storing duplicate data, enhancing data security.
Primary Key:
- A primary key is unique.
- A data table can only contain one primary key.
Foreign Key: Associate two tables.
Composite Key: use multiple columns as a single index key, usually used for composite indexes.
Install
Im lazy,see⬇️
SQL
Data Definition Language
- Obtain data in the database catalog
- Part of action queries
- CREATE, DROP, ALTER
Data Manipulation Language
- Add, modify, and remove rows from tables
- INSERT, UPDATE, DELETE
Data Query Language
- retrieve data from tables, determining how the data is presented to applications.
- SELECT, WHERE, ORDER BY, GROUP BY, HAVING
Transaction Processing Language
- Let all rows affected by DML update promptly
- BEGIN TRANSACTION, COMMIT, ROLLBACK
Cursor Control Language
Independent operations on one or more tables.
DECLARE CURSOR, FETCH INTO, UPDATE WHERE CURRENT
Data Control Language
- Determine individual user’s access to database objects
- GRANT, REVOKE
DDL
DB
1 | #create |
Table
1 | CREATE table table_name( |
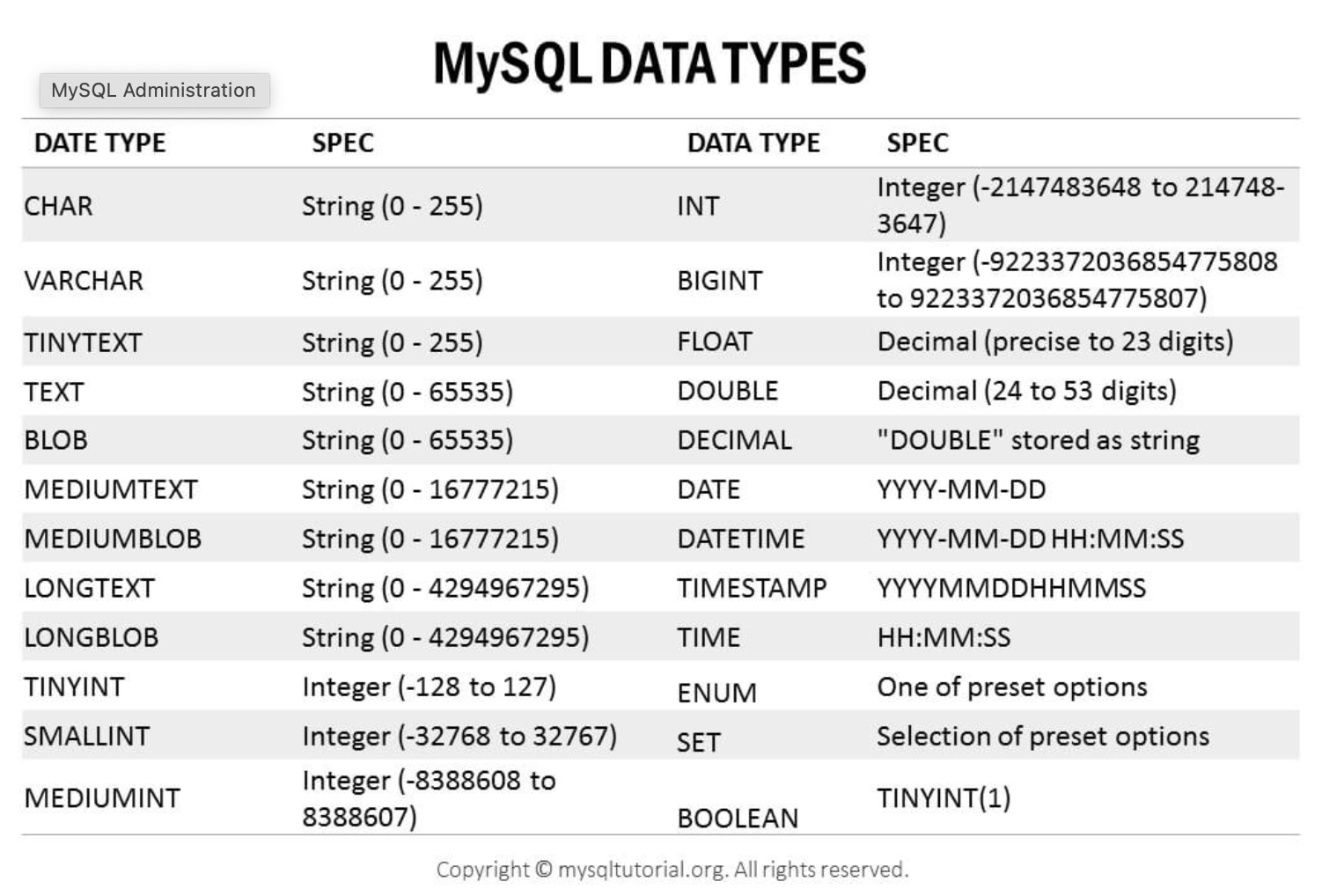
Show
1 | show tables; |
Create
1 | create table <NewDB> like <OldDB>; #Same Structure |
Delete
1 | drop table <dbName>; |
Alter
1 | alter table <dbName> add <columnName> datatype; #add |
Delete
1 | alter table <dbName> drop <columnName>; #delete column |
DML
Insert
1 | insert into <dbName> [column1,...,columnN] values(value1,...,valueN); |
Update
1 | update <dbName> set col1=val1,...,colN=valN [where conditionExpression]; |
Delete
1 | delete from <dbName> [where conditionExpression]; |
delte remove records
truncate removes the complete data from an existing table but not the table itself
DQL
Query
1 | select * from <dbName>; # query all the records, show all the cols |
Alias
1 | select <colName> as <aliasName> from <dbName>; |
Distinct
1 | select distinct col1,...,colN from <dbName>; |
Operation
<> unequal
In mysql could also use !=between … and … (Contain border)
in(val1,…, valN) Equal to the values
not in (val1, …, valN) not equal to the values
is, don’t use ‘’=’’ !!!!!!!!!!!!!
and, or ,not (yknow)
Like
Search for a specified pattern in a col
% : represent any amount of characters
_ : represent a single character
eg
1 | select * from people where name like 'a%' |
Sort
1 | select */cols from <dbName> [where conditionExpression] [order by <colName> [asc/desc]] |
asc: ascending,default
desc: descending
Group by
- Exhaustiveness
- Mutual exclusivity
1 | select */cols from <dbName> [group by cols [having conditionExpression]] |
WHERE
- Filter data before grouping
- Cannot use aggregate functions
HAVING
- Filter data after grouping
- Can use aggregate functions.
Aggregate Functions
count(colName)
sum(colName)
avg(colName) # Average
min(colName)
max(colName)
1 | select func(colName) from <dbName>; |
group_concat
Concatenate data from rows;
1 | select col1,...,colN group_concat ([distinct] col1 [order by clause] [separator str_val]) from <dbName> group by col2; |
Distinct: eleminate the repetition
order by: sort
separator: default is ,
with rollup
Add a new row at the bottom to record the total sum of all records in the columns
limit
1 | select * from <dbName> [limit offset, count]; |
offset: start from the offset, default 0
count: number of records to display
Fuzzy Query
%: any number of characters
_: single character, usually used to limit the length of the string
[]: any character in the brackets (like regex)
[^]: any character not in the brackets